Centering text is a common task in web development, and there are several ways to do it using HTML and CSS. Whether you’re designing a website, formatting content for an email, or simply experimenting with HTML code, centering text is a fundamental skill that ensures your content looks balanced and visually appealing.
In this article, we’ll explore the different methods for centering text in HTML, ranging from basic inline styles to more advanced CSS techniques. By the end, you’ll know how to center text both horizontally and vertically, making your content stand out in all the right ways.
1. Centering Text Using the <center>
Tag (Deprecated)
In earlier versions of HTML, the simplest way to center text was to use the <center>
tag. Here’s an example:
<center>This text is centered using the <center> tag.</center>
However, the <center>
tag is now deprecated in HTML5, meaning it’s no longer considered a best practice. Modern HTML and CSS provide more flexible and maintainable ways to center text, so it’s recommended to avoid using the <center>
tag in new projects.
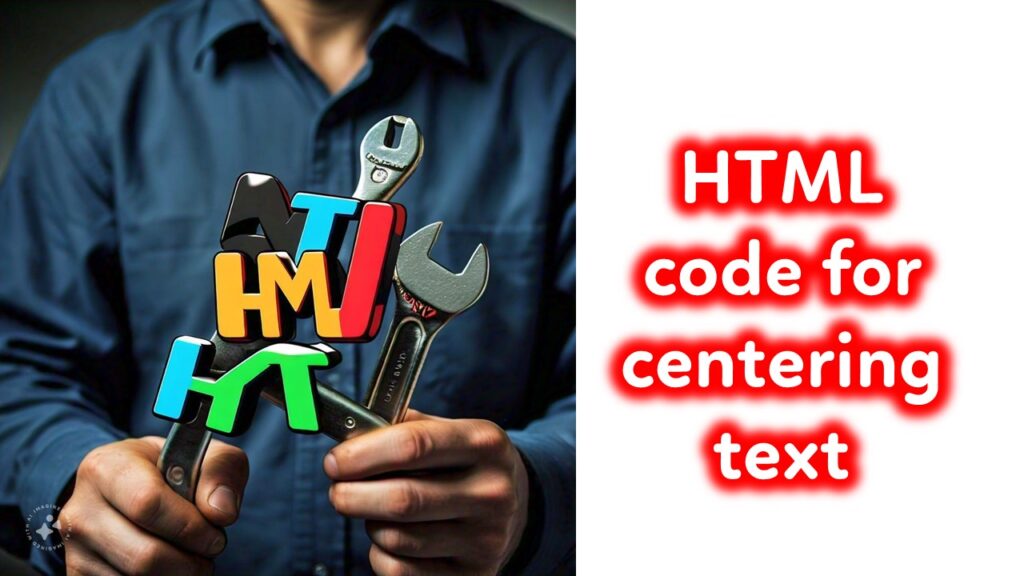
2. Centering Text with CSS (Modern Method)
To center text in modern web development, the preferred approach is to use CSS. There are several ways to achieve this, depending on the context. Let’s go over the most commonly used methods.
A. Centering Text Horizontally
The most basic and widely-used method for centering text horizontally is to apply the text-align
CSS property. Here’s how to do it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Centering Text Horizontally</title>
<style>
.center-text {
text-align: center;
}
</style>
</head>
<body>
<p class="center-text">This text is centered horizontally.</p>
</body>
</html>
In this example:
- The
text-align: center;
rule is applied to the paragraph element (<p>
). - This method centers the text inside its parent container (in this case, the body or a div).
B. Centering Text Vertically
Vertically centering text can be a bit more complex, depending on the structure of your HTML. A commonly used method is to utilize Flexbox.
Flexbox Method
Flexbox makes it easy to align content both horizontally and vertically. Here’s how to center text vertically and horizontally within a container:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Centering Text Vertically</title>
<style>
.center-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Set a height for the container */
}
</style>
</head>
<body>
<div class="center-container">
<p>This text is centered both vertically and horizontally.</p>
</div>
</body>
</html>
In this example:
display: flex;
creates a flexbox layout.justify-content: center;
horizontally centers the text.align-items: center;
vertically centers the text within the container.- The container is given a
height
of 100vh, which is 100% of the viewport height, ensuring the text is vertically centered on the screen.
C. Centering Text in a Div
If you’re working within a specific container (like a <div>
), you can combine the text-align
property with Flexbox for complete control. Here’s an example of centering text within a <div>
both horizontally and vertically:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Centering Text in a Div</title>
<style>
.center-div {
display: flex;
justify-content: center;
align-items: center;
height: 200px;
border: 2px solid black;
}
</style>
</head>
<body>
<div class="center-div">
<p>This text is centered inside the div.</p>
</div>
</body>
</html>
This example shows how Flexbox can easily center content within any block-level element, like a <div>
. The key properties here are justify-content
and align-items
, which ensure the text is centered in both directions.
3. Centering Text in a Grid Layout
Another modern approach to centering content is by using CSS Grid, which offers powerful tools for creating complex layouts, including text alignment. Here’s how to center text using Grid:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Centering Text with Grid</title>
<style>
.grid-container {
display: grid;
place-items: center;
height: 100vh;
}
</style>
</head>
<body>
<div class="grid-container">
<p>This text is centered using CSS Grid.</p>
</div>
</body>
</html>
Here, the place-items: center;
rule centers the text both horizontally and vertically within the grid container. Grid is similar to Flexbox but often more powerful for layout control, particularly in more complex designs.
4. Centering Text Inline (Using text-align
Directly)
For small projects or quick fixes, you can also apply text-align: center;
directly as an inline style within your HTML tag:
<p style="text-align: center;">This text is centered inline.</p>
While this works, it’s generally better to use CSS in an external stylesheet or in the <style>
tag for easier maintenance and cleaner code.
5. Centering Text in a Table Cell
When dealing with tables, centering text can be done using the text-align
property for horizontal centering and vertical-align
for vertical centering. Here’s an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Centering Text in a Table</title>
<style>
table {
width: 100%;
height: 200px;
border-collapse: collapse;
}
td {
text-align: center;
vertical-align: middle;
border: 1px solid black;
}
</style>
</head>
<body>
<table>
<tr>
<td>This text is centered in the table cell.</td>
</tr>
</table>
</body>
</html>
In this example, text-align: center;
centers the text horizontally within the table cell, while vertical-align: middle;
centers it vertically.
Conclusion
Centering text in HTML can be done using various methods, from the deprecated <center>
tag to modern, responsive approaches like Flexbox and CSS Grid. The best method depends on your layout requirements and how you want the text to behave across different screen sizes and devices.
Here’s a quick recap:
- For horizontal centering, use
text-align: center;
. - For vertical centering, use Flexbox or Grid.
- For more complex layouts or responsiveness, Flexbox and Grid provide powerful tools to ensure your content is centered effectively.
By using these modern HTML and CSS techniques, you’ll have complete control over how your text is aligned, ensuring a polished and professional look for your web content.
of course like your website but you have to check the spelling on several of your posts A number of them are rife with spelling issues and I in finding it very troublesome to inform the reality on the other hand I will certainly come back again